Create randomly duplicate objects in Maya with python script generated from chatGPT. I wish this functionality was a buit-in feature in Maya. Trust me, you are going to love it.
Even though Maya has a similar built-in feature “Duplicate Special“, it won’t given the same results, not even close. It lags in the randomness parameter. That command, it’s producess more or less perfect algorithmic results.
There is another built-in feature in Maya “Mash” procedural effects which can give similar results, but again not the same, with a lot of adjustments from our end although. You can take a glance of how powerful this tools is Creating a procedural earth map with MASH in Maya.
In this article, we are going to see how we can randomly duplicate 3D objects with ease. Plus it has a UI for easily access. Let’s just call this script, Random Diplicator.
This python script can be used with any geometry in Maya (polygonal, surface, curves).
With the following python script, we can specify:
- the numbers of copies through slider (50 max, but can be adjusted through the script)
- the size of the container that those duplicate objects will be generated (translate X, Y, Z)
- adjusting the random rotation of those generated objects (rotation X, Y, Z)
- adjusting the random scale of those generated objects (scale X, Y, Z)
- reset the settings
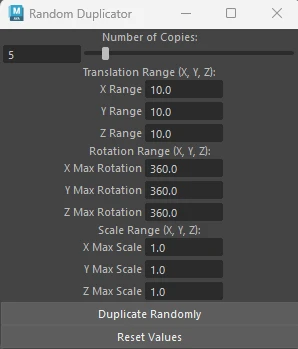
Randomly Duplicate Objects in Maya (Python Script):
As I said, i generated this script with the help of chatGPT. So the cretits goes to AI, since I am not a coder, although I have some basic knowledge of the Python programming language.
Python script for Random Duplicator (left-click on any of the prompts below to expand the script):
randomly duplicate objects in Maya (python script)
import maya.cmds as cmds
import random
def create_ui():
# Check if the window exists and delete if it does
if cmds.window("randomDupWin", exists=True):
cmds.deleteUI("randomDupWin")
# Create a new window
window = cmds.window("randomDupWin", title="Random Duplicator", widthHeight=(300, 400))
# Create a column layout
cmds.columnLayout(adjustableColumn=True)
# Slider to select number of copies
cmds.text(label="Number of Copies:")
num_copies_slider = cmds.intSliderGrp("numCopiesSlider", field=True, minValue=1, maxValue=50, value=5)
# Fields to input translation range
cmds.text(label="Translation Range (X, Y, Z):")
translate_x_field = cmds.floatFieldGrp("translateXField", numberOfFields=1, label="X Range", value1=10.0)
translate_y_field = cmds.floatFieldGrp("translateYField", numberOfFields=1, label="Y Range", value1=10.0)
translate_z_field = cmds.floatFieldGrp("translateZField", numberOfFields=1, label="Z Range", value1=10.0)
# Fields to input rotation range
cmds.text(label="Rotation Range (X, Y, Z):")
rotate_x_field = cmds.floatFieldGrp("rotateXField", numberOfFields=1, label="X Max Rotation", value1=360.0)
rotate_y_field = cmds.floatFieldGrp("rotateYField", numberOfFields=1, label="Y Max Rotation", value1=360.0)
rotate_z_field = cmds.floatFieldGrp("rotateZField", numberOfFields=1, label="Z Max Rotation", value1=360.0)
# Fields to input scale range
cmds.text(label="Scale Range (X, Y, Z):")
scale_x_field = cmds.floatFieldGrp("scaleXField", numberOfFields=1, label="X Max Scale", value1=1.0)
scale_y_field = cmds.floatFieldGrp("scaleYField", numberOfFields=1, label="Y Max Scale", value1=1.0)
scale_z_field = cmds.floatFieldGrp("scaleZField", numberOfFields=1, label="Z Max Scale", value1=1.0)
# Button to trigger duplication
cmds.button(label="Duplicate Randomly", command=lambda x: duplicate_randomly(
num_copies_slider, translate_x_field, translate_y_field, translate_z_field,
rotate_x_field, rotate_y_field, rotate_z_field,
scale_x_field, scale_y_field, scale_z_field
))
# Reset button to reset all values
cmds.button(label="Reset Values", command=lambda x: reset_values(
num_copies_slider, translate_x_field, translate_y_field, translate_z_field,
rotate_x_field, rotate_y_field, rotate_z_field,
scale_x_field, scale_y_field, scale_z_field
))
# Show the window
cmds.showWindow(window)
def duplicate_randomly(slider, tx_field, ty_field, tz_field, rx_field, ry_field, rz_field, sx_field, sy_field, sz_field):
# Get the number of copies from the slider
num_copies = cmds.intSliderGrp(slider, query=True, value=True)
# Get the translation ranges
translate_x = cmds.floatFieldGrp(tx_field, query=True, value1=True)
translate_y = cmds.floatFieldGrp(ty_field, query=True, value1=True)
translate_z = cmds.floatFieldGrp(tz_field, query=True, value1=True)
# Get the rotation ranges
rotate_x = cmds.floatFieldGrp(rx_field, query=True, value1=True)
rotate_y = cmds.floatFieldGrp(ry_field, query=True, value1=True)
rotate_z = cmds.floatFieldGrp(rz_field, query=True, value1=True)
# Get the scale ranges
scale_x = cmds.floatFieldGrp(sx_field, query=True, value1=True)
scale_y = cmds.floatFieldGrp(sy_field, query=True, value1=True)
scale_z = cmds.floatFieldGrp(sz_field, query=True, value1=True)
# Get the selected objects
selected_objects = cmds.ls(selection=True)
if not selected_objects:
cmds.warning("No objects selected. Please select an object to duplicate.")
return
# Duplicate the selected object randomly
for _ in range(num_copies):
for obj in selected_objects:
# Duplicate the object
new_obj = cmds.duplicate(obj)[0]
# Apply random transformations
random_translate = [
random.uniform(-translate_x, translate_x),
random.uniform(-translate_y, translate_y),
random.uniform(-translate_z, translate_z)
]
random_rotate = [
random.uniform(-rotate_x, rotate_x),
random.uniform(-rotate_y, rotate_y),
random.uniform(-rotate_z, rotate_z)
]
random_scale = [
random.uniform(1.0, scale_x),
random.uniform(1.0, scale_y),
random.uniform(1.0, scale_z)
]
cmds.setAttr(f"{new_obj}.translate", *random_translate)
cmds.setAttr(f"{new_obj}.rotate", *random_rotate)
cmds.setAttr(f"{new_obj}.scale", *random_scale)
def reset_values(slider, tx_field, ty_field, tz_field, rx_field, ry_field, rz_field, sx_field, sy_field, sz_field):
# Reset the slider
cmds.intSliderGrp(slider, edit=True, value=5)
# Reset translation fields
cmds.floatFieldGrp(tx_field, edit=True, value1=10.0)
cmds.floatFieldGrp(ty_field, edit=True, value1=10.0)
cmds.floatFieldGrp(tz_field, edit=True, value1=10.0)
# Reset rotation fields
cmds.floatFieldGrp(rx_field, edit=True, value1=360.0)
cmds.floatFieldGrp(ry_field, edit=True, value1=360.0)
cmds.floatFieldGrp(rz_field, edit=True, value1=360.0)
# Reset scale fields
cmds.floatFieldGrp(sx_field, edit=True, value1=1.0)
cmds.floatFieldGrp(sy_field, edit=True, value1=1.0)
cmds.floatFieldGrp(sz_field, edit=True, value1=1.0)
# Run the UI
create_ui()
How to use this script:
Open up the Script Editor in Maya, by going to Windows – General Editors – Script Editor. Create a Python tab. Paste the above lines of script, in the Script Editor in Maya. Select all the lines (ctrl + A) for shortcut, and press ctrl + Enter, to execute the script.
Our new Random Diplicator UI window appears.
Select any 3D object in Maya and, after adjusting all the settings you need from this window, hit the Duplicate Randomly button at the bottom.
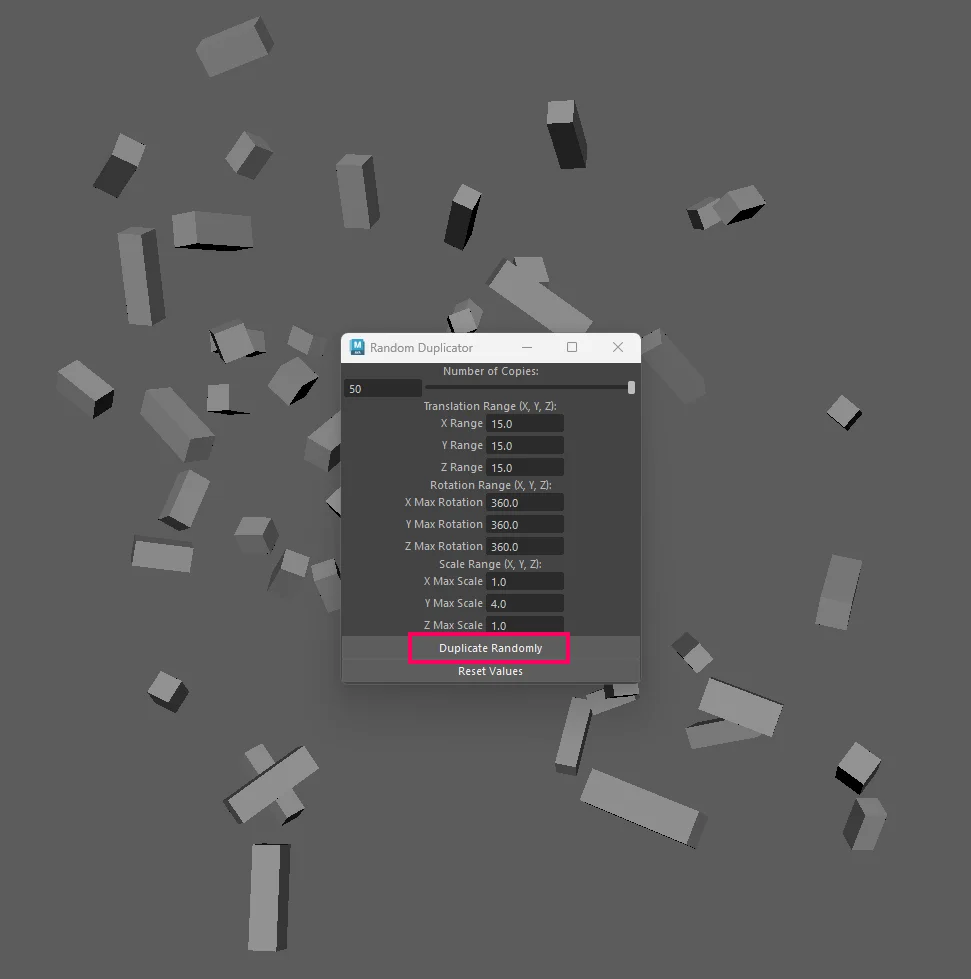
Don’t forget to put the script into a shelf in Maya for easy access, in case you use it a lot into your projects.
Increase the number of copies:
At this stage of the script, the maximun numbers of copies you can generate is 50. If you want to increse it you can change the maxValue=50 of the following line of the code in the script editor, at any number you want:
num_copies_slider = cmds.intSliderGrp(“numCopiesSlider”, field=True, minValue=1, maxValue=50, value=5)
That was it, I hope you enjoy the script.
My step-by-step approach prompts with chatGPT:
Left-click on any of the prompts below to expand the script.
create a python script in Maya with UI to randomly duplicate an object with a slider attribute of the number of copys
import maya.cmds as cmds
import random
def create_ui():
# Check if the window exists and delete if it does
if cmds.window("randomDupWin", exists=True):
cmds.deleteUI("randomDupWin")
# Create a new window
window = cmds.window("randomDupWin", title="Random Duplicator", widthHeight=(300, 150))
# Create a column layout
cmds.columnLayout(adjustableColumn=True)
# Slider to select number of copies
cmds.text(label="Number of Copies:")
num_copies_slider = cmds.intSliderGrp("numCopiesSlider", field=True, minValue=1, maxValue=50, value=5)
# Button to trigger duplication
cmds.button(label="Duplicate Randomly", command=lambda x: duplicate_randomly(num_copies_slider))
# Show the window
cmds.showWindow(window)
def duplicate_randomly(slider):
# Get the number of copies from the slider
num_copies = cmds.intSliderGrp(slider, query=True, value=True)
# Get the selected objects
selected_objects = cmds.ls(selection=True)
if not selected_objects:
cmds.warning("No objects selected. Please select an object to duplicate.")
return
# Duplicate the selected object randomly
for _ in range(num_copies):
for obj in selected_objects:
# Duplicate the object
new_obj = cmds.duplicate(obj)[0]
# Apply random transformations
random_translate = [random.uniform(-10, 10) for _ in range(3)]
random_rotate = [random.uniform(0, 360) for _ in range(3)]
cmds.setAttr(f"{new_obj}.translate", *random_translate)
cmds.setAttr(f"{new_obj}.rotate", *random_rotate)
# Run the UI
create_ui()
add three values translate X, Y, Z to act as a container for the duplicate objects
import maya.cmds as cmds
import random
def create_ui():
# Check if the window exists and delete if it does
if cmds.window("randomDupWin", exists=True):
cmds.deleteUI("randomDupWin")
# Create a new window
window = cmds.window("randomDupWin", title="Random Duplicator", widthHeight=(300, 200))
# Create a column layout
cmds.columnLayout(adjustableColumn=True)
# Slider to select number of copies
cmds.text(label="Number of Copies:")
num_copies_slider = cmds.intSliderGrp("numCopiesSlider", field=True, minValue=1, maxValue=50, value=5)
# Fields to input translation range
cmds.text(label="Translation Range (X, Y, Z):")
translate_x_field = cmds.floatFieldGrp("translateXField", numberOfFields=1, label="X Range", value1=10.0)
translate_y_field = cmds.floatFieldGrp("translateYField", numberOfFields=1, label="Y Range", value1=10.0)
translate_z_field = cmds.floatFieldGrp("translateZField", numberOfFields=1, label="Z Range", value1=10.0)
# Button to trigger duplication
cmds.button(label="Duplicate Randomly", command=lambda x: duplicate_randomly(num_copies_slider, translate_x_field, translate_y_field, translate_z_field))
# Show the window
cmds.showWindow(window)
def duplicate_randomly(slider, tx_field, ty_field, tz_field):
# Get the number of copies from the slider
num_copies = cmds.intSliderGrp(slider, query=True, value=True)
# Get the translation ranges
translate_x = cmds.floatFieldGrp(tx_field, query=True, value1=True)
translate_y = cmds.floatFieldGrp(ty_field, query=True, value1=True)
translate_z = cmds.floatFieldGrp(tz_field, query=True, value1=True)
# Get the selected objects
selected_objects = cmds.ls(selection=True)
if not selected_objects:
cmds.warning("No objects selected. Please select an object to duplicate.")
return
# Duplicate the selected object randomly
for _ in range(num_copies):
for obj in selected_objects:
# Duplicate the object
new_obj = cmds.duplicate(obj)[0]
# Apply random transformations
random_translate = [
random.uniform(-translate_x, translate_x),
random.uniform(-translate_y, translate_y),
random.uniform(-translate_z, translate_z)
]
random_rotate = [random.uniform(0, 360) for _ in range(3)]
cmds.setAttr(f"{new_obj}.translate", *random_translate)
cmds.setAttr(f"{new_obj}.rotate", *random_rotate)
# Run the UI
create_ui()
add another three values roation X, Y, Z to controll the maximn rotation for the duplicate objects
import maya.cmds as cmds
import random
def create_ui():
# Check if the window exists and delete if it does
if cmds.window("randomDupWin", exists=True):
cmds.deleteUI("randomDupWin")
# Create a new window
window = cmds.window("randomDupWin", title="Random Duplicator", widthHeight=(300, 300))
# Create a column layout
cmds.columnLayout(adjustableColumn=True)
# Slider to select number of copies
cmds.text(label="Number of Copies:")
num_copies_slider = cmds.intSliderGrp("numCopiesSlider", field=True, minValue=1, maxValue=50, value=5)
# Fields to input translation range
cmds.text(label="Translation Range (X, Y, Z):")
translate_x_field = cmds.floatFieldGrp("translateXField", numberOfFields=1, label="X Range", value1=10.0)
translate_y_field = cmds.floatFieldGrp("translateYField", numberOfFields=1, label="Y Range", value1=10.0)
translate_z_field = cmds.floatFieldGrp("translateZField", numberOfFields=1, label="Z Range", value1=10.0)
# Fields to input rotation range
cmds.text(label="Rotation Range (X, Y, Z):")
rotate_x_field = cmds.floatFieldGrp("rotateXField", numberOfFields=1, label="X Max Rotation", value1=360.0)
rotate_y_field = cmds.floatFieldGrp("rotateYField", numberOfFields=1, label="Y Max Rotation", value1=360.0)
rotate_z_field = cmds.floatFieldGrp("rotateZField", numberOfFields=1, label="Z Max Rotation", value1=360.0)
# Button to trigger duplication
cmds.button(label="Duplicate Randomly", command=lambda x: duplicate_randomly(num_copies_slider, translate_x_field, translate_y_field, translate_z_field, rotate_x_field, rotate_y_field, rotate_z_field))
# Show the window
cmds.showWindow(window)
def duplicate_randomly(slider, tx_field, ty_field, tz_field, rx_field, ry_field, rz_field):
# Get the number of copies from the slider
num_copies = cmds.intSliderGrp(slider, query=True, value=True)
# Get the translation ranges
translate_x = cmds.floatFieldGrp(tx_field, query=True, value1=True)
translate_y = cmds.floatFieldGrp(ty_field, query=True, value1=True)
translate_z = cmds.floatFieldGrp(tz_field, query=True, value1=True)
# Get the rotation ranges
rotate_x = cmds.floatFieldGrp(rx_field, query=True, value1=True)
rotate_y = cmds.floatFieldGrp(ry_field, query=True, value1=True)
rotate_z = cmds.floatFieldGrp(rz_field, query=True, value1=True)
# Get the selected objects
selected_objects = cmds.ls(selection=True)
if not selected_objects:
cmds.warning("No objects selected. Please select an object to duplicate.")
return
# Duplicate the selected object randomly
for _ in range(num_copies):
for obj in selected_objects:
# Duplicate the object
new_obj = cmds.duplicate(obj)[0]
# Apply random transformations
random_translate = [
random.uniform(-translate_x, translate_x),
random.uniform(-translate_y, translate_y),
random.uniform(-translate_z, translate_z)
]
random_rotate = [
random.uniform(-rotate_x, rotate_x),
random.uniform(-rotate_y, rotate_y),
random.uniform(-rotate_z, rotate_z)
]
cmds.setAttr(f"{new_obj}.translate", *random_translate)
cmds.setAttr(f"{new_obj}.rotate", *random_rotate)
# Run the UI
create_ui()
include another thee values scale X, Y, Z to control the maximun scale on those axes for the duplicate objects
import maya.cmds as cmds
import random
def create_ui():
# Check if the window exists and delete if it does
if cmds.window("randomDupWin", exists=True):
cmds.deleteUI("randomDupWin")
# Create a new window
window = cmds.window("randomDupWin", title="Random Duplicator", widthHeight=(300, 400))
# Create a column layout
cmds.columnLayout(adjustableColumn=True)
# Slider to select number of copies
cmds.text(label="Number of Copies:")
num_copies_slider = cmds.intSliderGrp("numCopiesSlider", field=True, minValue=1, maxValue=50, value=5)
# Fields to input translation range
cmds.text(label="Translation Range (X, Y, Z):")
translate_x_field = cmds.floatFieldGrp("translateXField", numberOfFields=1, label="X Range", value1=10.0)
translate_y_field = cmds.floatFieldGrp("translateYField", numberOfFields=1, label="Y Range", value1=10.0)
translate_z_field = cmds.floatFieldGrp("translateZField", numberOfFields=1, label="Z Range", value1=10.0)
# Fields to input rotation range
cmds.text(label="Rotation Range (X, Y, Z):")
rotate_x_field = cmds.floatFieldGrp("rotateXField", numberOfFields=1, label="X Max Rotation", value1=360.0)
rotate_y_field = cmds.floatFieldGrp("rotateYField", numberOfFields=1, label="Y Max Rotation", value1=360.0)
rotate_z_field = cmds.floatFieldGrp("rotateZField", numberOfFields=1, label="Z Max Rotation", value1=360.0)
# Fields to input scale range
cmds.text(label="Scale Range (X, Y, Z):")
scale_x_field = cmds.floatFieldGrp("scaleXField", numberOfFields=1, label="X Max Scale", value1=2.0)
scale_y_field = cmds.floatFieldGrp("scaleYField", numberOfFields=1, label="Y Max Scale", value1=2.0)
scale_z_field = cmds.floatFieldGrp("scaleZField", numberOfFields=1, label="Z Max Scale", value1=2.0)
# Button to trigger duplication
cmds.button(label="Duplicate Randomly", command=lambda x: duplicate_randomly(
num_copies_slider, translate_x_field, translate_y_field, translate_z_field,
rotate_x_field, rotate_y_field, rotate_z_field,
scale_x_field, scale_y_field, scale_z_field
))
# Show the window
cmds.showWindow(window)
def duplicate_randomly(slider, tx_field, ty_field, tz_field, rx_field, ry_field, rz_field, sx_field, sy_field, sz_field):
# Get the number of copies from the slider
num_copies = cmds.intSliderGrp(slider, query=True, value=True)
# Get the translation ranges
translate_x = cmds.floatFieldGrp(tx_field, query=True, value1=True)
translate_y = cmds.floatFieldGrp(ty_field, query=True, value1=True)
translate_z = cmds.floatFieldGrp(tz_field, query=True, value1=True)
# Get the rotation ranges
rotate_x = cmds.floatFieldGrp(rx_field, query=True, value1=True)
rotate_y = cmds.floatFieldGrp(ry_field, query=True, value1=True)
rotate_z = cmds.floatFieldGrp(rz_field, query=True, value1=True)
# Get the scale ranges
scale_x = cmds.floatFieldGrp(sx_field, query=True, value1=True)
scale_y = cmds.floatFieldGrp(sy_field, query=True, value1=True)
scale_z = cmds.floatFieldGrp(sz_field, query=True, value1=True)
# Get the selected objects
selected_objects = cmds.ls(selection=True)
if not selected_objects:
cmds.warning("No objects selected. Please select an object to duplicate.")
return
# Duplicate the selected object randomly
for _ in range(num_copies):
for obj in selected_objects:
# Duplicate the object
new_obj = cmds.duplicate(obj)[0]
# Apply random transformations
random_translate = [
random.uniform(-translate_x, translate_x),
random.uniform(-translate_y, translate_y),
random.uniform(-translate_z, translate_z)
]
random_rotate = [
random.uniform(-rotate_x, rotate_x),
random.uniform(-rotate_y, rotate_y),
random.uniform(-rotate_z, rotate_z)
]
random_scale = [
random.uniform(1.0, scale_x),
random.uniform(1.0, scale_y),
random.uniform(1.0, scale_z)
]
cmds.setAttr(f"{new_obj}.translate", *random_translate)
cmds.setAttr(f"{new_obj}.rotate", *random_rotate)
cmds.setAttr(f"{new_obj}.scale", *random_scale)
# Run the UI
create_ui()
include a reset button in the UI to reset values translation 10, rotation 360, scale 1
import maya.cmds as cmds
import random
def create_ui():
# Check if the window exists and delete if it does
if cmds.window("randomDupWin", exists=True):
cmds.deleteUI("randomDupWin")
# Create a new window
window = cmds.window("randomDupWin", title="Random Duplicator", widthHeight=(300, 400))
# Create a column layout
cmds.columnLayout(adjustableColumn=True)
# Slider to select number of copies
cmds.text(label="Number of Copies:")
num_copies_slider = cmds.intSliderGrp("numCopiesSlider", field=True, minValue=1, maxValue=50, value=5)
# Fields to input translation range
cmds.text(label="Translation Range (X, Y, Z):")
translate_x_field = cmds.floatFieldGrp("translateXField", numberOfFields=1, label="X Range", value1=10.0)
translate_y_field = cmds.floatFieldGrp("translateYField", numberOfFields=1, label="Y Range", value1=10.0)
translate_z_field = cmds.floatFieldGrp("translateZField", numberOfFields=1, label="Z Range", value1=10.0)
# Fields to input rotation range
cmds.text(label="Rotation Range (X, Y, Z):")
rotate_x_field = cmds.floatFieldGrp("rotateXField", numberOfFields=1, label="X Max Rotation", value1=360.0)
rotate_y_field = cmds.floatFieldGrp("rotateYField", numberOfFields=1, label="Y Max Rotation", value1=360.0)
rotate_z_field = cmds.floatFieldGrp("rotateZField", numberOfFields=1, label="Z Max Rotation", value1=360.0)
# Fields to input scale range
cmds.text(label="Scale Range (X, Y, Z):")
scale_x_field = cmds.floatFieldGrp("scaleXField", numberOfFields=1, label="X Max Scale", value1=1.0)
scale_y_field = cmds.floatFieldGrp("scaleYField", numberOfFields=1, label="Y Max Scale", value1=1.0)
scale_z_field = cmds.floatFieldGrp("scaleZField", numberOfFields=1, label="Z Max Scale", value1=1.0)
# Button to trigger duplication
cmds.button(label="Duplicate Randomly", command=lambda x: duplicate_randomly(
num_copies_slider, translate_x_field, translate_y_field, translate_z_field,
rotate_x_field, rotate_y_field, rotate_z_field,
scale_x_field, scale_y_field, scale_z_field
))
# Reset button to reset all values
cmds.button(label="Reset Values", command=lambda x: reset_values(
num_copies_slider, translate_x_field, translate_y_field, translate_z_field,
rotate_x_field, rotate_y_field, rotate_z_field,
scale_x_field, scale_y_field, scale_z_field
))
# Show the window
cmds.showWindow(window)
def duplicate_randomly(slider, tx_field, ty_field, tz_field, rx_field, ry_field, rz_field, sx_field, sy_field, sz_field):
# Get the number of copies from the slider
num_copies = cmds.intSliderGrp(slider, query=True, value=True)
# Get the translation ranges
translate_x = cmds.floatFieldGrp(tx_field, query=True, value1=True)
translate_y = cmds.floatFieldGrp(ty_field, query=True, value1=True)
translate_z = cmds.floatFieldGrp(tz_field, query=True, value1=True)
# Get the rotation ranges
rotate_x = cmds.floatFieldGrp(rx_field, query=True, value1=True)
rotate_y = cmds.floatFieldGrp(ry_field, query=True, value1=True)
rotate_z = cmds.floatFieldGrp(rz_field, query=True, value1=True)
# Get the scale ranges
scale_x = cmds.floatFieldGrp(sx_field, query=True, value1=True)
scale_y = cmds.floatFieldGrp(sy_field, query=True, value1=True)
scale_z = cmds.floatFieldGrp(sz_field, query=True, value1=True)
# Get the selected objects
selected_objects = cmds.ls(selection=True)
if not selected_objects:
cmds.warning("No objects selected. Please select an object to duplicate.")
return
# Duplicate the selected object randomly
for _ in range(num_copies):
for obj in selected_objects:
# Duplicate the object
new_obj = cmds.duplicate(obj)[0]
# Apply random transformations
random_translate = [
random.uniform(-translate_x, translate_x),
random.uniform(-translate_y, translate_y),
random.uniform(-translate_z, translate_z)
]
random_rotate = [
random.uniform(-rotate_x, rotate_x),
random.uniform(-rotate_y, rotate_y),
random.uniform(-rotate_z, rotate_z)
]
random_scale = [
random.uniform(1.0, scale_x),
random.uniform(1.0, scale_y),
random.uniform(1.0, scale_z)
]
cmds.setAttr(f"{new_obj}.translate", *random_translate)
cmds.setAttr(f"{new_obj}.rotate", *random_rotate)
cmds.setAttr(f"{new_obj}.scale", *random_scale)
def reset_values(slider, tx_field, ty_field, tz_field, rx_field, ry_field, rz_field, sx_field, sy_field, sz_field):
# Reset the slider
cmds.intSliderGrp(slider, edit=True, value=5)
# Reset translation fields
cmds.floatFieldGrp(tx_field, edit=True, value1=10.0)
cmds.floatFieldGrp(ty_field, edit=True, value1=10.0)
cmds.floatFieldGrp(tz_field, edit=True, value1=10.0)
# Reset rotation fields
cmds.floatFieldGrp(rx_field, edit=True, value1=360.0)
cmds.floatFieldGrp(ry_field, edit=True, value1=360.0)
cmds.floatFieldGrp(rz_field, edit=True, value1=360.0)
# Reset scale fields
cmds.floatFieldGrp(sx_field, edit=True, value1=1.0)
cmds.floatFieldGrp(sy_field, edit=True, value1=1.0)
cmds.floatFieldGrp(sz_field, edit=True, value1=1.0)
# Run the UI
create_ui()
If you found this script interested and useful, you may also like this one as well: Select Random Faces in Maya with Python Script from ChatGPT.